使用Linux Socket编程实现一个Dormitory Reservation System.
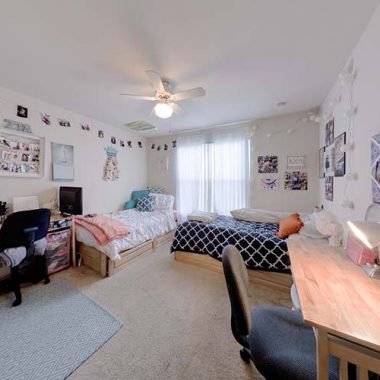
Objective
The objective of this assignment is to familiarize you with UNIX socket programming. It is an individual assignment and no collaborations are allowed. Any cheating will result in an automatic F in the course (not just in the assignment). If you have any doubts/questions, post your questions on D2L. You must discuss all project related issues on the Piazza discussion forum. We will give those who actively help others out by answering questions on the Piazza discussion forum up to 10 bonus points.
Problem Statement
Dormitory Reservation Systems are key for managing student housing efficiently. They help organize room bookings, make finding and booking rooms easier, speed up the booking process, and give useful information for making decisions. These systems make it easier and quicker for students to find housing and for staff to understand which rooms are most wanted. This helps manage room assignments better and respond faster to student needs, making sure everyone finds a place to stay.
Security is very important too. It’s essential to make sure the system is safe by requiring usernames and passwords. This stops people who are not students from booking rooms and keeps housing available for actual students. So, building a safe, reliable, easy-to-use online booking system is very important for our dormitory to work well.
For this project, you’ll make a simple dormitory booking system. We’ll divide the dormitory into three types of rooms: Single Rooms, Double Rooms, and Suites, to keep things organized.
Students can look up if the type of room they want is available and book it if it is. They use a client interface to reach the main dormitory server, which then connects with the specific section server corresponding to a specific room type. Each section server has information on the rooms it handles. The main server also checks the user’s identity.
- Client: The system features include checking if rooms are available and booking them, with two types of clients: Guest and Member.
- Members: can log in, search for room availability, and book a room.
- Book a room, which will then decrease the room availability by 1 in the backend server’s data structure (this change is only in the data structure and not written back to the input file).
- Members’ usernames and passwords are stored in a file named “members.txt.”
- Guests can only search for room availability.
- Users who skip the password input by pressing “Enter” will be treated as a Guest.
- Members: can log in, search for room availability, and book a room.
- Main Server (ServerM): Verifies the identity of the students and coordinates with the backend servers.
- Backend Servers (Single (S), Double (D), Suite (U)): Store the information of a specific room type.
This dormitory reservation system aims to provide a seamless and efficient process for students seeking on-campus housing, ensuring a positive experience from search to reservation.
Source Code Files
Your implementation should include the source code files described below, for each component of the system.
- Client: The name of this piece of code must be client.c or client.cc or client.cpp (all small letters) and the header file (if you have one; it is not mandatory) must be called client.h (all small letters).
- serverM (Main Server): You must name your code file: serverM.c or serverM.cc or serverM.cpp (all small letters except ‘M’). Also, you must include the corresponding header file (if you have one; it is not mandatory) serverM.h (all small letters except ‘M’).
- Backend Servers S, D, U: You are required to create three distinct files, choosing from the following naming conventions: ServerS.c, ServerS.cc, ServerS.cpp or ServerD.c, ServerD.cc, ServerD.cpp or ServerU.c, ServerU.cc, ServerU.cpp. The filename must utilize one of these formats, substituting “#” with the specific server identifier (either “A” or “B”) to reflect the server it represents, resulting in filenames like serverA.c, serverA.cc, serverA.cpp, serverB.c, serverB.cc, or serverB.cpp (note that the name should be entirely in lowercase except for the letter replacing “#”). If available, you should also include a corresponding header file named server#.h, adhering to the same naming rule for the “#” replacement. This ensures a clear, organized naming structure for your code and its associated header file, if any.
Note: You are not allowed to use one executable for all four servers (i.e. a “fork” based implementation).
Input Files
- member.txt: contains encrypted usernames and passwords. This file should only be accessed by the Main server.
- single.txt: contains single room information categorized in roomcode, and number of the available rooms. Different categories are separated by a comma. This file should only be accessed by the Backend Server S.
- double.txt: contains double room information categorized in roomcode, and number of the available rooms. Different categories are separated by a comma. This file should only be accessed by the Backend Server D.
- suite.txt: contains suite room information categorized in roomcode, and number of the available rooms. Different categories are separated by a comma. This file should only be accessed by the Backend Server U.
Note: member_unencrypted.txt is the unencrypted version of member.txt, which is provided for your reference to enter a valid username and password. It should NOT be touched by any servers!
Detailed Explanation
Phase 1: Bootup
Please refer to the “Process Flow” section to start your programs in the order of the main serverM, server S, server D, server U, and two Clients. Your programs must start in this order. Each of the servers and the clients have boot-up messages that must be printed on the screen. Please refer to the on-screen messages section for further information.
When three backend servers (server S, server D, and server U) are up and running, each backend server should read the corresponding input file (single.txt, double.txt, and suites.txt) and store the information in a certain data structure. You can choose any data structure that accommodates your needs. After storing all the data, server S, server D, and server U should then send all the room statuses they have to the main server via UDP over the port mentioned in the PORT NUMBER ALLOCATION section. Since the room statuses are unique, the main server will maintain a list of room statuses corresponding to each backend server. In the following phases, you have to make sure that the correct backend server is being contacted by the main server for corresponding room statuses. You should print correct on-screen messages onto the screen for the main server and the backend servers indicating the success of these operations as described in the “ON-SCREEN MESSAGES” section.
After the servers are booted up and the required room statuses are transferred from the backend servers to the main server, the client will be started. Once the client boots up and the initial bootup messages are printed, the client waits for the user to check the authentication, login, and enter the room layout code.
Please check Table 3 and 4 Client on-screen messages for the on-screen message of different events You should store the above room statuses. Once you have the book statuses list stored in your backend server and send the book code list of each backend server to the main server, you can consider phase 1 of the project to be completed. You can proceed to phase 2.
Phase 2: Log in and confirmation
In this phase, the client will be asked to enter the username and password on the terminal. There are two types of clients: guest and member. A member can be authenticated by the Dormitory Reservation System by inputting the member’s username and password. The client will encrypt this information and forward this request to the Main server. The Main server would have all the encrypted credentials (both username and password would be encrypted) of the registered users. Still, it would not have any information about the encryption scheme. The information about the encryption scheme would only be present on the client side. A guest can input the username while skipping the password input, but a guest can only query the room status but cannot reserve a room.
The encryption scheme for member authentication would be as follows:
- Offset each character and/or digit by 3.
- character: cyclically alphabetic (A-Z, a-z) update for overflow
- digit: cyclically 0-9 update for overflow
- The scheme is case-sensitive.
- Special characters (including spaces and/or the decimal point) will not be encrypted or changed.
A few examples of encryption are given below:
Example | Original Text | Encrypted Text |
---|---|---|
#1 | Welcome to EE450! | Zhofrph wr HH783! |
#2 | 199xyz@$ | 422abc@$ |
#3 | 0.27#& | 3.50#& |
Constraints:
- The username will be of lower case characters (5~50 chars).
- The password will be case sensitive (5~50 chars)
Phase 2A
A member client sends the authentication request to the main server over TCP connection. Upon running the client using the following command, the user will be prompted to enter the username and password. This unencrypted information will be encrypted at client side and then sent to the main server over TCP. A guest client can skip the password authentication and directly login.
./client (Please refer to the on-screen messages)
Please enter the username: <unencrypted_username>
Please enter the password ("Enter" to skip for guests): <unencrypted_password>
Phase 2B
Main server receives the encrypted username and password from the client. ServerM sends the result of the authentication request to the client over a TCP connection. If the login information was not correct/found:
./client (Please refer to the on-screen messages)
Please enter the username: <unencrypted_username>
Please enter the password: <unencrypted_password>
Failed login. Invalid username/password
After the successful login:
Welcome member/guest <username>!
Please enter the room layout code: <roomcode>
Multiple clients
In phase 2, Main server will have to receive requests from both the clients. For a server to receive requests from several clients at the same time, the function fork() should be used for the creation of a new process. Fork() function is used for creating a new process, which is called child process, which runs concurrently with the process that makes the fork() call (parent process).
For a TCP server, when an application is listening for stream-oriented connections from other hosts, it is notified of such events and must initialize the connection using accept(). After the connection with the client is successfully established, the accept() function returns a non-zero descriptor for a socket called the child socket. The server can then fork off a process using fork() function to handle connection on the new socket and go back to waiting on the original socket. Note that the socket that was originally created, that is the parent socket, is going to be used only to listen to the client requests, and it is not going to be used for communication between client and Main server. Child sockets that are created for a parent socket have the identical well-known port number IP address at the server side, but each child socket is created for a specific client. Through using the child socket with the help of fork(), the server can handle the two clients without closing any one of the connections.
Phase 3: Forwarding request to Backend Servers
Phase 3A: Query
Both a member client and a guest client can query the current statues of a specific room layout. Upon user input of a room code for a type and layout, the client is responsible for transmitting the request to the server M (the Main server) via a TCP connection. The server M parses the received roomcode to determine the appropriate destination server for request forwarding. Specifically, when the roomcode commences with “S,” the request must be routed to Server S. Similarly, if the roomcode initiates with “D,” the request is directed to Server D. In the event that the roomcode originates with “U,” the request must be forwarded to Server U. All the valid book codes are eligible for forwarding to their respective servers from the Server M via a UDP connection.
RoomCode from Client | Source Server | Destination Server |
---|---|---|
S146 | Server M | Server S |
D111 | Server M | Server D |
U211 | Server M | Server U |
A111 | Server M | None |
Phase 3B: Reservation
Only the member client can reserve a room. Each server will have a dedicated database file. This file should be read only once at server startup to ensure that if a user reserves a room, the corresponding book’s inventory count is updated accurately in the respective data structure and must not be overwritten by reading the database file over and over. The updated room number will be printed on the member client screen.
If a guest is requesting a reservation, a “permission denied” prompt will show on screen.
Phase 4: Reply
The corresponding room type server will check its input file and find the count of the requested roomcode. If the count is greater than 0, then the respective server will reply to the main server using UDP - “The requested room is available”. And if the count of the room is 0, then the server will reply to the main server using UDP - “The requested room is not available”. It is also possible that the roomcode entered by the client is not there in the system, in that case, the server will respond with a message - “Not able to find the room layout”.
For a reservation request, after sending the reply to the main server the room type server will decrement the count of the corresponding roomcode by 1 so that when a client requests a book a second time, the availability is updated and correct. And at last, the main server will print the onscreen message and will forward the reply from the room type server to the client using TCP. And the client will print the on screen message which gives the availability of the requested roomcode.
See ON-SCREEN MESSAGES table for more details.
Extra credit
In this section, we will enhance the security of this system by applying more powerful encryption algorithms. You are encouraged to search the Internet for any existing security encryption/decryption algorithms to substitute the simple character shifting method in Phase 2, or you can even create some complicated methods as long as the transmitted message is not in plaintext. If you use symmetric or asymmetric encryption with public/private keys, you can assume they are known by the host and servers, and the keys can be hardcoded in the code.
To get full credit for this part, you are required to explain the algorithm with all details as well as show an example of the original text and encrypted text in the Readme.txt file (you can create an extra credit section), and you also need to provide clear instructions to tell graders how to compile and execute the system using upgraded encryption algorithms. (For example, you can add an argument to programs indicating which encryption protocol you are using, as shown in the sample code below. Then in Makefile, you can create another entry “make extra” to compile and run the program using this more secure and complicated encryption/decryption algorithm.)
Process Flow/Sequence of Operations
- Your project grader will start the servers in this sequence: serverM, serverS, serverD, serverU, and two Clients in 6 different terminals.
- Once all the ends are started, the servers and clients should be continuously running unless stopped manually by the grader or meet certain conditions as mentioned before.
Required Port Number Allocation
The ports to be used by the clients and the servers for the exercise are specified in the following table (Major points will be lost if the port allocation is not as per the below description)
Example Output to Illustrate Output Formatting
Server S Terminal:
The Server S is up and running using UDP on port 41319.
The Server S has sent the room status to the main server.
The Server S received an availability request from the main server.
Room S146 is available.
The Server S finished sending the response to the main server.
Server D Terminal:
The Server D is up and running using UDP on port 42319.
The Server D has sent the room status to the main server.
The Server D received an availability request from the main server.
Room D111 is not available.
The Server D finished sending the response to the main server.
Server U Terminal:
The Server U is up and running using UDP on port 43319.
The Server U has sent the room status to the main server.
The Server U received a reservation request from the main server.
Successful reservation. The count of Room U211 is now 1.
The Server U finished sending the response and the updated room status to the main server.
Main Server Terminal:
The main server is up and running.
The main server has received the room status from Server S using UDP over port 44319.
The main server has received the room status from Server D using UDP over port 44319.
The main server has received the room status from Server U using UDP over port 44319.
The main server received the authentication for james using TCP over port 45319.
The main server sent the authentication result to the client.
The main server has received the availability request on Room S146 from james using TCP over port 45319.
The main server sent a request to Server S.
The main server received the response from Server D using UDP over port 44319.
The main server sent the availability information to the client.
The main server has received the availability request on Room D111 from james using TCP over port 45319.
The main server sent a request to Server D.
The main server received the response from Server D using UDP over port 44319.
The main server sent the availability information to the client.
The main server has received the reservation request on Room U211 from james using TCP over port 45319.
The main server sent a request to Server U.
The main server received the response and the updated room status from Server U using UDP over port 44319.
The room status of Room U211 has been updated.
The main server sent the reservation result to the client.
Client Terminal
Client is up and running.
Please enter the username: james
Please enter the password: 2kAnsa7s james sent an authentication request to the main server.
Welcome member james!
Please enter the room code: S146
Would you like to search for the availability or make a reservation? (Enter "Availability" to search for the availability or Enter "Reservation" to make a reservation ): Availability james sent an availability request to the main server.
The client received the response from the main server using TCP over port [port number].
The requested room is available.
-----Start a new request-----
Please enter the room code: D111
Would you like to search for the availability or make a reservation? (Enter "Availability" to search for the availability or Enter "Reservation" to make a reservation ): Availability james sent an availability request to the main server.
The client received the response from the main server using TCP over port [port number].
The requested room is not available.
-----Start a new request----
Please enter the room code: U211
Would you like to search for the availability or make a reservation? (Enter "Availability" to search for the availability or Enter "Reservation" to make a reservation ): Reservation james sent a reservation request to the main server.
The client received the response from the main server using TCP over port [port number].
Congratulation! The reservation for Room U211 has been made.
-----Start a new request----
Please enter the room code: [Ctrl C]
Submission Files
Submission File and Folder Structure:
Your submission should have the following folder structure and the files (the examples are of .cpp, but it can be .c files as well):
- ee450_lastname_firstname_uscusername.tar.gz
- ee450_lastname_firstname_uscusername
- client.cpp
- serverM.cpp
- serverS.cpp
- serverD.cpp
- serverU.cpp
- Makefile
- readme.txt (or) readme.md
- Any additional header files
- ee450_lastname_firstname_uscusername
The grader will extract the tar.gz file, and will place all the input data files in the same directory as your source files. The executable files should also be generated in the same directory as your source files. So, after testing your code, the folder structure should look something like this:
- ee450_lastname_firstname_uscusername
- client.cpp
- serverM.cpp
- serverS.cpp
- serverD.cpp
- serverU.cpp
- Makefile
- readme.txt (or) readme.md
- client
- serverM
- serverS
- serverD
- serverU
- member.txt
- single.txt
- double.txt
- suites.txt
- Any additional header files
Note that in the above example, the input data files (member.txt, single.txt, double.txt and suites.txt) will be manually placed by the grader, while the ‘make all’ command should generate the executable files.
Assumptions
- You have to start the processes in this order: serverM, serverS, serverD, serverU, and client. If you need to have more code files than the ones that are mentioned here, please use meaningful names and all small letters and mention them all in your README file.
- You are allowed to use blocks of code from Beej’s socket programming tutorial (Beej’s guide to network programming) in your project. However, you need to cite the copied part in your code. Any signs of academic dishonesty will be taken very seriously.
- When you run your code, if you get the message “port already in use” or “address already in use”, please first check to see if you have a zombie process. If you do not have such zombie processes or if you still get this message after terminating all zombie processes, try changing the static UDP or TCP port number corresponding to this error message (all port numbers below 1024 are reserved and must not be used). If you have to change the port number, please do mention it in your README file and provide reasons for it.
Requirements
- Do not hardcode the TCP or UDP port numbers that are to be obtained dynamically.
Refer to Table Port Number Allocation to see which ports are statically defined and which ones are dynamically assigned. Use getsockname() function to retrieve the locallybound port number wherever ports are assigned dynamically as shown below. - The host name must be hard coded as “localhost” or “127.0.0.1” in all codes.
- Your client, the backend servers and the main server should keep running and be waiting for another request until the TAs terminate them by Ctrl+C. If they terminate before that, you will lose some points for it.
- All the naming conventions and the on-screen messages must conform to the previously mentioned rules.
- You are not allowed to pass any parameter or value or string or character as a commandline argument except what is already described in the project document.
- All the on-screen messages must conform exactly to the project description. You should not add anymore on-screen messages. If you need to do so for the debugging purposes, you must comment out all of the extra messages before you submit your project.
- Please do remember to close the socket and tear down the connection once you are done using that socket.
Programming platform and environment
- All your submitted code MUST work well on the provided virtual machine Ubuntu.
- All submissions will only be graded on the provided Ubuntu. TAs/Graders won’t make any updates or changes to the virtual machine. It’s your responsibility to make sure your code works well on the provided Ubuntu. “It works well on my machine” is not an excuse.
- Your submission MUST have a Makefile. Please follow the requirements in the following “Submission Rules” section