对题目给出的编程语法,用Java代写一个Recursive descent parser.
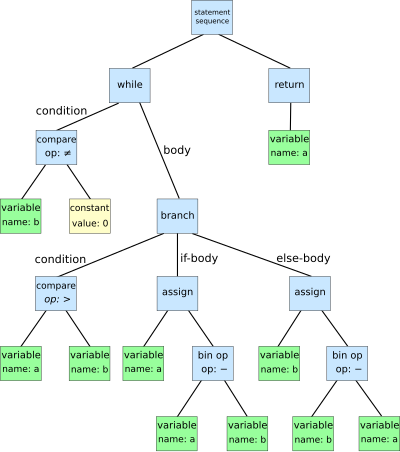
Problem 1
Write a recursive descent parser for the language generated by the grammar:
S → ε
→ E_LIST
E LIST → EXPR E_TAIL
E TAIL → ε
→ E_LIST
EXPR → S_EXPR
S_EXPR → ANDOP S_TAIL
S_TAIL → ε
→ ′|′ S EXPR
ANDOP → RELOP A_TAIL
A_TAIL → ε
→ ′&′ ANDOP
RELOP → TERM R_TAIL
R_TAIL → ε
→ ′<′ RELOP
→ ′>′ RELOP
→ ′=′ RELOP
→ ′#′ RELOP
TERM → FACT T_TAIL
T_TAIL → ε
→ ′+′ TERM
→ ′−′ TERM
FACT → VALUE F_TAIL
F_TAIL → ε
→ ′∗′ FACT
→ ′/′ FACT
VALUE → LIST
→ UNARY
→ LITERAL
→ ′(′EXPR′)′
→ SYMBOL
LIST → ′[′ARGS′]′
UNARY → ′−′ VALUE
→ ′!′ VALUE
ARGS → ε
→ EXPR A_LIST
A_LIST → ε
→ ′,′ EXPRA_LIST
SYMBOL → symbol
LITERAL→ integer
→ string
→ ′true′
→ ′false′
→ ′nil′
The terminal int denotes an integer, string denotes a double quoted string, e.g., “hello world” and the terminal symbol denotes a symbol, e.g., myVar.
You should use the scanner that you built (or the solution provided) as the front end to the parser. That is, the scanner will take input from stdin and generate tokens, which the parser will then use. See the provided test cases for examples of input.
The output of your parser should be the list of productions being applied (in order) as the parser parses the input. If the token stream to your parser represents a valid program, the parser should terminate after all productions have been applied (and printed). If the parser cannot finish parsing (the token stream does not represent a valid program, the last line of output should be: Syntax Error
The format of the productions that are to printed by the parser as they are being applied are of the form: LHS -> RHS
where the LHS is a variable as shown in Figure 1 and the RHS consists of terminals and and variables, where terminals are depicted in single quotes, except for integers, strings, and symbols.
- symbol is denoted by symbol(x) where x is the symbol name, e.g.,
symbol(myVar)
- integer is denoted by int(v) where v is the integer value, e.g.,
int(42)
- string is denoted by string(s) where s is the string in double quotes, e.g.,
string("Hello World")
For example, the outputs for the following the program
A set of test cases is provided on Brightspace in the file tests_5.zip The sample solution takes about 160 lines of code (not counting the scanner).
You may implement your parser in any language that you wish, but it must be runnable on the bluenose server. Since the choice of language is up to you, you must provide a standard script called runme.sh to run your parser. For example the script for a Java solution looks like:1
2
3
4
5
6
# if your implementation requires compilation include the command here
javac SplatParser.java
# Command to run your program
java SplatParser
Please submit your code, along with a runme.sh script via brightspace.
Problem 2
Give a PDA (Pushdown Automata) that recognizes the language
L={σ∈{x,y,z}∗ |2|σ|x =|σ|y ∨2|σ|y =|σ|z}
You can choose whether your PDA accepts by empty stack or final state, but make sure you clearly note, which acceptance is assumed.
Assignments are due by 9:00am on the due date. Assignments must be submitted electronically via Brightspace. Please submit zip file of the code and a PDF for the written work. You can do your work on paper and then scan in and submit the assignment.