练习JavaScript中异步编程 (Asynchronous Programming) 的使用方法。
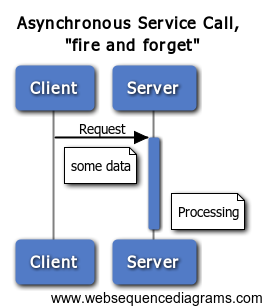
Purpose
The purpose of this lab is to familiarize yourself with asynchronous programming in JavaScript, as well as using modules from the Node.js Package Manager npm.
For this lab, you must use the async/await keywords (not Promises). You will also be using axios, which is a HTTP client for Node.js. After you run npm init with to create your package.json, you can install and save axios to it with npm i axios. You should check your package.jsondependencies to ensure that axios is present:
In addition, you must have error checking for the arguments of all your functions. If an argument fails error checking, you should throw a string describing which argument was wrong, and what went wrong.
Network JSON Data
You will be downloading JSON files from the following GitHub Gists:
- people.json
- weather.json
- work.json
For every function you write, you will download the necessary JSONs with axios. Here is an example of how to do so:1
2
3
4async function getPeople() {
const { data } = await axios.get('https://.../people.json')
return data // this will be the array of people
}
Functions
getPersonById(index)
This will return the person at a specified index within the people.json array.
You must check:
- That the index exists as is of proper type
- The index is within bounds
1 | await getPersonById(42) // Returns: "Brew Peat" |
lexIndex(index)
For this function, you must get the sorted lexographic (aka alphabetical order) of all the people (in people.json) by their last name. Then, return the person’s full name at the index specified by the argument index.
You must check:
- That the index exists as is of proper type
- The index is within bounds
1 | await lexIndex(2) // Returns: "Dermot Abberley" |
firstNameMetrics()
Using just the first names of all the people in people.json, collect and return the following metrics:1
2
3
4
5
6
7{
totalLetters: sum of all the letters in all the firstNames,
totalVowels: sum of all the vowels in all the firstNames,
totalConsonants: sum of all the consonants in all the firstNames,
longestName: the longest firstName in the list,
shortestName: the shortest firstName in the list
}
This function does not take any arguments.
shouldTheyGoOutside(firstName, lastName)
Given a first and last name, find the person in the people.jsonarray, then, if they are found, get their zip code and find the temperature for their location in weather.json. If the temperature is greater than or equal to 34 degrees, the person will go outside. You can assume that the weather data for the zip codes exist.
Make sure to follow the example string below exactly.
You must check:
- That both parameters exist and are of the proper type.
- That the person specified exists in the people.json array.
1 | await shouldTheyGoOutside("Scotty", "Barajaz") // Returns "Yes, Scotty should go outside." |
whereDoTheyWork(firstName, lastName)
Given a first and last name, find the person in the people.jsonarray, then, if they are found, get their SSN and find out where they work from work.json. Then, print their full name, company, job title and if they will be fired. You can assume that the data corresponding to a person’s SSN always exists in work.json.
Make sure to follow the example string below exactly.
You must check:
- That both parameters exist and are of the proper type.
- That the person specified exists in the people.json array.
1 | whereDoTheyWork("Demetra", "Durrand") // Returns: "Demetra Durrand - Nuclear Power Engineer at Buzzshare. They will be fired." |
findTheHacker(ip)
Someone is hacking company records, and your boss found their IP Address. Use your async JS skills with people.json and work.json to find the hacker!
You must check:
- That the ip address specified exists and is of proper type.
1 | findTheHacker("79.222.167.180") // Returns: "Robert Herley is the hacker!" |
Requirements
- Write each function in the specified file and export the function so that it may be used in other files.
- Ensure to properly error check for different cases such as arguments existing and of the proper type as well as throw. if anything is out of bounds such as invalid array index or negative numbers for different operations.
- Submit all files (including package.json) in a zip with your name in the following format: LastName_FirstName.zip.
- Make sure to save any npm packages you use to you package.json
- DO NOT submit a zip containing your node_modules folder