使用p5.js实现Web端的基本的几何图形的绘制以及交互功能。
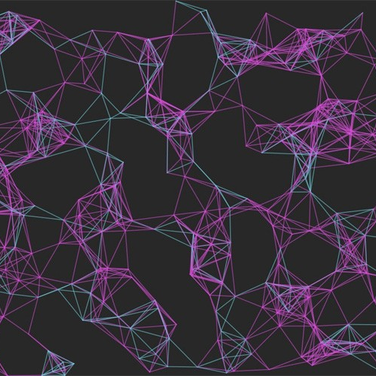
Learning outcomes
You should be able to
- Use p5.js to develop code
- Write a class to encapsulate information about objects
- Use multiple definitions of distance
- Color individual pixels of a sketch
- Write code that responds to mouse and keyboard interactions
- Write an algorithm that colors pixels based on their distances from a given set of random points
In the following diagram
- The black points are a set of n randomly generated points. Let’s call this set P consisting of p1, p2, , pn. We will refer to each of these points as a “site”.
- Each pixel in the sketch is colored based on which of the sites it is closest to, using Euclidean distance to measure distance. So, the set of all the points that are closest to site p1 have the same color. All points that are closest to site p2 have the same color, and so on. So, for example, if you are standing anywhere in the red wedge, then you are closer to the site (represented by the black point) in the red wedge than you are to any of the other sites.
Assignment
- Using p5.js, create a sketch that generates a diagram like the one above:
- Create a class that stores both the location and the color for a site. Then, create an array of sites.
- Include a variable at the top of your source code that makes it easy to change the initial number of sites. Your code should work well with any number of sites (as few as 1 site, and as many as several hundred).
- Use a canvas size of whatever you want, but each side should be at least 400 and no more than 1000. Distribute the points randomly within the sketch. Your sketch should work even if I were to change the size of canvas.
- If your sketch is too slow, you could use something smaller than 400 while testing. But change it back to 400 or more before submitting.
- Color each pixel based on which of the sites in the array it is closest to, initially using euclidean distance as the measure (note that p5.js’s dist() method uses Euclidean distance). You should use a random color for each region. You could experiment with this. For example, random shades of red, or grey, or pastel colors, or something else.
- Use set() to color the pixels (as you did in the first lab)
- Remember to use updatePixels() after the loop that sets all the pixel colors.
- You should also display the black dots representing the sites.
- Display these last, after updatePixels(), so that they appear on top of your colored regions.
- You could use a color other than black for the sites. The goal is to make them stand out from the rest of the points.
- After the above is working, add the following modifications to your code:
- Pressing the right and left arrow keys should cycle between euclidean distance, taxicab distance, and Chebyshev (chessboard) distance.
- See the keyPressed() reference page for help on detecting keys.
- An elegant solution to this would be to create an array of distance functions (see your first lab for how to create that array). For example, you could begin with this:
let distFunctions = [];
And then add your distance functions to the array. See the first lab.
- As you move the mouse around the sketch, the region that your mouse is in should be highlighted. When the mouse moves out of that region, the color should return to its normal color.
- Clicking on the sketch with the right mouse button should add a new site at the location where the mouse was clicked, and the diagram should be redrawn. All the previous regions should still use the same colors they were using.
- Clicking on the sketch with the left mouse button should remove the site that the mouse is in, and the diagram should be redrawn. All the remaining regions should still use the same colors they were using.
- Do not remove the last site: if there is only one site remaining, don’t do anything if the user clicks the left mouse button.
- Pressing the right and left arrow keys should cycle between euclidean distance, taxicab distance, and Chebyshev (chessboard) distance.
Submit the URL of the EDIT version of your sketch to Canvas by the due date.
p5js notes: (see the p5js reference page)
- Be sure you are logged in to p5js while coding.
- Ctrl-Shift-F (or Cmd-Shift-F) will tidy up your source code. Please use it.
- p5js allows for creating separate class files (using separate javascript files), but you can also just define it in the same file with the rest of your code, as we have done in class. See p5js’s reference page for class. If you decide to write a separate javascript file for a separate class, you will need to update the html file to include that additional file.
- Use p5js’s set() function to change the color of a pixel. It is much faster than using point(). But, you could use point() to draw the sites.
- Call updatePixels() after the loop that sets the pixel colors. If you call updatePixels() inside the loop, you will have a sketch that runs too slowly, making both you and your instructor very sad.
- In p5js, color is a data type. This line of code creates and stores a color:
let c = color(255, 0, 0);
- Javascript has a splice() function for removing array elements.
Scoring
- See assignment details for the scoring.
- Deductions may be made for code that does not follow standard programming styles, poor decomposition into functions, too much repeated code, and so on