使用C++的Loop,实现简单的应用小程序,不能使用高级语法。
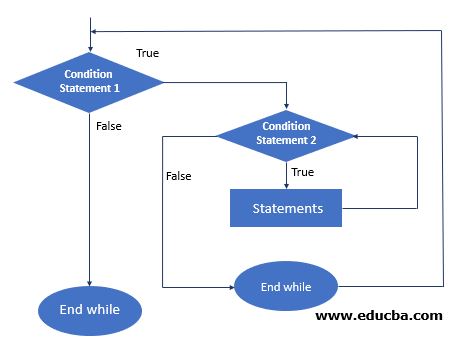
Requirement
- You must turn in your program listing and output for each program set. Each program set must have your student name, student ID, and program set number/description. Late homework will not be accepted for whatever reasons you may have.
For this homework, you are to submit your Program sets to Canvas under Homework 3 link- a. Name your files: HW3_PS1_ lastname_firstinitial.c for Program Set 1 and HW3_PS2_ lastname_firstinitial.c for PS2 and so on. PS means program set. If there are two program sets you will submit two files one for each program set. Example if your name is Joe Smith then the filename will be HW3_PS1_smith_j.c
- b. You must submit your homework by the deadline at the specified upload link on Canvas under homework 3. If the deadline is past, Program Sets will not be graded. Homework submitted via email attachment, comment in Canvas, Canvas message, or by any other method is not accepted and will be given a zero for no submission.
- c. if you do not follow instructions on file naming provided in this section you will receive a zero for the question you did not correctly name the file.
- d. It is your responsibility to check if your homework is properly submitted to Canvas.
- Please format your output properly, for example all dollar amounts should be printed with 2 decimal places. Make sure that your output values are correct (check the calculations).
- Use only the ‘tools’ in the topics we covered up till loops only. No
#include<math.h]>, #include<iostream>, #include<stdlib.h>, functions, arrays, structs, scanf("%[^\n]%*c",varname)
and any topics not covered from lesson 1 to lesson 32 except to declare strings char strVar[20]. A zero grade will be given if any of the listed is used.
Grading
If a program does not compile the program set will receive a zero score. If the program compiles and run but does not have proper declaration of variables, syntax, logic, and displays the correct output (specified in the sample test runs) with proper formatting as specified in the question, the program set will receive a zero score. Each program set must compile and must run correctly with proper declaration of variables, syntactically, logically, and display the correct output (given in the sample test runs) as specified then you will receive the full points for that question. Then points will be deducted for not having proper:
- a. Comments 1 pt deducted for each infraction
- Title Banner –Your name, description at the beginning of each program set.
- Short description of the what each section or function of your codes do.
- b. Consistency/Readability 1 pt deducted for each infraction
- Spacing(separate each section of codes with a blank line
- Proper Indentation for loops and if/switch statements
- Proper naming of variables no a, b, c - use descriptive and mnemonics)
- c. Required elements 1 pt deducted for each infraction
- proper formatting for output when specified
- all monetary values must be in 2 decimal places when specified in sample test runs
- d. Use only ‘tools’ in the topics that have been covered in class. For example, for this homework we have not covered functions, arrays, structs. So, if you use a function, array, struct you will receive a zero for the whole program set. See also item 3 above.
- e. Output (you must provide the specified number of test runs or your program set will receive a zero score)
- to be displayed at the end of the program listing(codes) and commented
- if no output(test runs) is provided for your uploaded file, a zero will be given for that program set.
- must have the number of test runs as specified in each program set.
- must use the data for test runs when they are provided for you in the question.
USE ONLY THE TOOLS YOU HAVE BEEN TAUGHT IN CLASS ONLY(Lesson 1 to Lesson 32), IF YOU USE ANYTHING WE HAVE NOT COVERED YET YOU WILL RECEIVE A ZERO FOR THAT PROGRAM SET (eg functions, arrays, structs)
The break, continue and goto C commands are not allowed to be used. A zero will be given if your program contains a break, continue or goto command. The break command is allowed only as part of the switch statement.
Program Set 1
Write a C program to calculate salary raise for employees.
If salary is between $ 0 < $ 30000 the rate is 7.0%
If salary is between $ 30000 <= $ 40000 the rate is 5.5%
If salary is greater than $ 40000 the rate is 4.0%
- Let the user enter salary. Allow the user to enter as many salaries as the user wishes until the user enters a negative salary to quit for example -1(sample test run 1). User can also decide to quit immediately after starting the program(sample test run 2). Pick the proper loop.
- Calculate the raise, new salary, total salary, total raise, and total new salary.
- Sample input and output (leftmost column in blue is user input):
Your output of 5 test runs must be exactly the same format as specified above. Use the data provided above from the sample test run 1 and 2. Your test run 2 should enter the salary of -1 as first value, your program should exit. Provide your own data for the rest of the 3 test runs using different numbers.
Program Set 2
- Using the same information as in program set 1. This program will first ask the user to how many salaries do you want to enter. Your program will then execute the exact number of salaries the user wanted to enter. Pick the proper loop. You cannot use the same loop as Program Set 1. Using the same loop as Program set 1 will result in a zero for Program Set 2
- Sample input and output (leftmost column is user input in blue)
Your output of 3 test runs must be exactly the same format as specified above. Use the data provided above for sample test run 1. Provide your own data for the rest of the 2 test runs.